A bitmap created from R.drawable.icon will be passed from one activity (AndroidPassingBitmap) to another activity (AndroidReceiveBitmap).
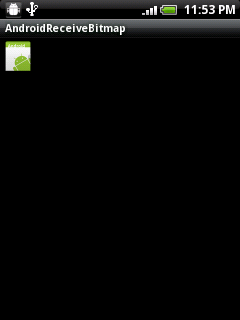
Modify the activity AndroidPassingBitmap.java, simple create a bitmap and pass to the second activity.
/AndroidPassingBitmap/src/com/AndroidPassingBitmap/AndroidPassingBitmap.java
?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package com.AndroidPassingBitmap;
import android.app.Activity;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
public class AndroidPassingBitmap extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.main);
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.icon);
Intent intent = new Intent();
intent.setClass(AndroidPassingBitmap. this , AndroidReceiveBitmap. class );
intent.putExtra( "Bitmap" , bitmap);
startActivity(intent);
}
}
|
Create a new activity to retrieve and display the bitmap.
/workspace/AndroidPassingBitmap/src/com/AndroidPassingBitmap/AndroidReceiveBitmap.java
?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package com.AndroidPassingBitmap;
import android.app.Activity;
import android.graphics.Bitmap;
import android.os.Bundle;
import android.widget.ImageView;
public class AndroidReceiveBitmap extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
Bitmap bitmap = (Bitmap) this .getIntent().getParcelableExtra( "Bitmap" );
setContentView(R.layout.newimage);
ImageView viewBitmap = (ImageView)findViewById(R.id.bitmapview);
viewBitmap.setImageBitmap(bitmap);
}
}
|
Create the layout of the second activity.
/workspace/AndroidPassingBitmap/res/layout/newimage.xml
?
1
2
3
4
5
6
7
8
9
10
11
12
13
|
<? xml version = "1.0" encoding = "utf-8" ?>
android:orientation = "vertical"
android:layout_width = "fill_parent"
android:layout_height = "fill_parent"
>
< ImageView
android:id = "@+id/bitmapview"
android:layout_width = "wrap_content"
android:layout_height = "wrap_content"
/>
</ LinearLayout >
|
Update AndroidManifest.xml to include the second activity in the package.
/workspace/AndroidPassingBitmap/AndroidManifest.xml
?
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
<? xml version = "1.0" encoding = "utf-8" ?>
package = "com.AndroidPassingBitmap"
android:versionCode = "1"
android:versionName = "1.0" >
< application android:icon = "@drawable/icon" android:label = "@string/app_name" >
< activity android:name = ".AndroidPassingBitmap"
android:label = "@string/app_name" >
< intent-filter >
< action android:name = "android.intent.action.MAIN" />
< category android:name = "android.intent.category.LAUNCHER" />
</ intent-filter >
</ activity >
< activity android:name = ".AndroidReceiveBitmap"
android:label = "AndroidReceiveBitmap" >
</ activity >
</ application >
< uses-sdk android:minSdkVersion = "4" />
</ manifest >
|